วิธีการดึงข้อมูล API ด้วยการใช้ axios
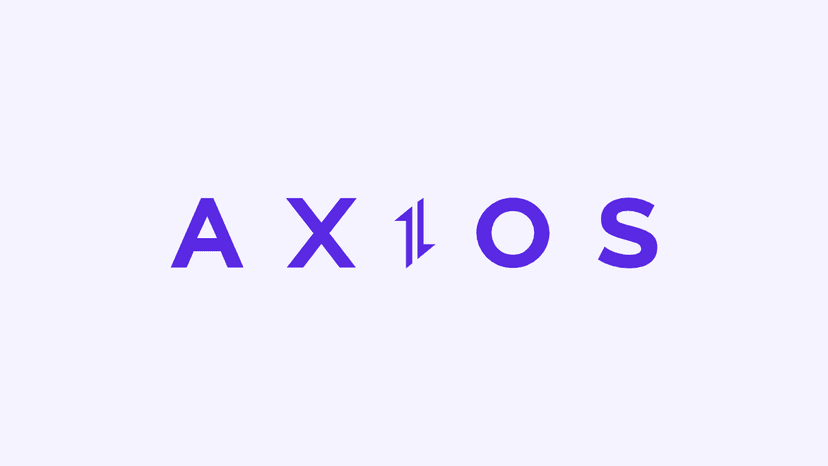
ต้องบอกว่า axios เป็น HTTP Client Library ตัวนึงที่คนนิยมใช้งานมากๆ เผลอๆ จะมากกว่า Fetch API ที่เป็น global module ของ Browser ซะอีก (รวมถึงฝั่ง Node.js ที่เพิ่งจะ built-in support ใน v18.0) วันนี้เลยมาเขียนบทความการใช้งาน axios ในแบบคร่าวๆ ไว้ครับ จริงๆ เรียกว่าสรุปและอ่านจาก Docs มากกว่า 🤣
Axios คืออะไร?
axios เป็น Promise based HTTP Client Library เพื่อทำการดึงข้อมูล ส่งข้อมูล และเชื่อมต่อกับ API โดยสามารถใช้งานได้ทั้งผ่าน Browser และ Node.js ซึ่ง Features หลักๆ ที่น่าสนใจของ axios คือ:
- รองรับ Promise API
- การแปลงข้อมูล
reauest
และresponse
- รองรับการ cancel request
- ทำ Intercept headers ได้
- ป้องกัน XSRF
- รองรับ TypeScript (จริงๆ ต้องบอกว่าเป็นมาตรฐานไปแล้ว Library ไหนไม่รองรับน่าจะแปลกกว่า)
Browser Support
![]() | ![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|---|
Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | 11 ✔ |
การติดตั้ง
เราสามารถใช้งาน axios ได้ทั้งผ่าน Browser หรือ Node.js ก็ได้
ติดตั้งด้วย NPM หรือ Yarn:
npm install axios# yarn add axios
หรือถ้าใช้งานผ่าน Browser ก็แทรกไปใน HTML ผ่าน CDN ได้เลย
1<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
ตัวอย่างการใช้งาน
ถ้าเราใช้งานแบบ CommonJS เวลา import ด้วย require()
เราจะใช้แบบด้านล่างนี้ เพื่อให้ Editor มันสามารถที่จะ support Autocomplete / Intellisense
1const axios = require('axios').default
แบบ ES Module
1import axios from 'axios'
GET Request
ตัวอย่างการใช้ GET
1const url = 'https://your-api-server'2
3axios4 .get(url)5 .then((response) => {6 // handle success7 console.log(response)8 })9 .catch((error) => {10 // handle errors11 })
ใช้งาน GET แบบส่ง Params
1const url = 'https://your-api-server/users?id=12345'2
3axios4 .get(url)5 .then((response) => {6 // handle success7 console.log(response)8 })9 .catch((error) => {10 // handle errors11 })
ซึ่งจะมีค่าเท่ากับแบบนี้ (เอา query params ออกจาก URL แล้วใช้ options
ส่งเป็น args ไปใน axios.get()
)
1const url = 'https://your-api-server/users'2
3const options = {4 params: {5 id: 123456 }7}8
9axios10 .get(url, options)11 .then((response) => {12 // handle success13 console.log(response)14 })15 .catch((error) => {16 // handle errors17 })
async/await
เราสามารถที่จะใช้การเขียนแบบ async/await ได้ เช่นกัน
1async function getUser() {2 try {3 const response = await axios.get('/your-api')4 console.log(response)5 } catch (error) {6 console.error(error)7 }8}
POST Request แบบ JSON
การส่ง POST Request แบบ JSON ใช้ การส่ง payload
เป็น args ตัวที่ 2 แบบนี้
1const payload = { title: 'Hello Axios' }2axios.post('/posts', payload)
โดยที่ default ตัว payload จะเป็น JSON และก็ Content-Type
จะเป็น application/json
POST Request แบบ application/x-www-form-urlencoded
เราสามารถส่ง POST Request แบบ application/x-www-form-urlencoded
ได้นอกเหนือจาก JSON โดยการใช้ URLSearchParams (ตัวนี้ไม่ได้รองรับทุก Browser นะ)
1const params = new URLSearchParams({ title: 'Hello Axios' })2axios.post('/posts', params)
POST Request แบบ multipart/form-data
การส่งแบบ multipart/form-data
เราจะใช้ FormData ใน Browser เราสามารถเรียกใช้ FormData API ได้ ส่วนถ้าเป็น Node.js ต้องใช้ Library เพิ่มเติม เช่น form-data
1const formData = new FormData()2formData.append('title', 'Hello Axios')3
4axios.post('/posts', formData)
ถ้าเป็น Node.js จะใช้ form-data
library
1const FormData = require('form-data')2const form = new FormData()3
4axios.post('/posts', form)
Auto Serialization to FormData
ตัว Axios ก็ยังรองรับการ Serialize JSON เป็น FormData
ให้อัตโนมัติ ถ้า Content-Type
เป็น multipart/form-data
เช่น
1import axios from 'axios'2
3axios4 .post(5 '/posts',6 { title: 'Hello Axios' },7 {8 headers: {9 'Content-Type': 'multipart/form-data'10 }11 }12 )13 .then(({ data }) => console.log(data))
Method ของ Axios
นอกจากนี้ ตัว axios ยังรองรับ HTTP Method เป็นชื่อเดียวกับ function เลย ตัวอย่าง
1axios.get(url)2
3axios.post(url, payload)4
5axios.put(url, payload)6
7axios.patch(url, payload)8
9axios.delete(url)
การ Custom Instance
เราสามารถ custom instance เพื่อกำหนดค่า config เองได้ เช่น กำหนด headers
หรือกำหนด baseURL
หรือ timeout
เป็นต้น
1// Set config defaults when creating the instance2const instance = axios.create({3 baseURL: 'https://api.example.com',4 headers: {5 'My-Custom-Header': 'custom-header'6 }7 timeout: 50008});9
10instance.defaults.headers.common['Authorization'] = 'token';
ตัวอย่างการ set token กรณีมีต้อง require token ในบาง Request แบบคร่าวๆ
1const instance = axios.create()2
3instance.interceptors.request.use(4 (config) => {5 // get token from cookies / session / localStorage6 const token = getSessionToken()7 if (token) {8 config.headers['x-access-token'] = token9 }10 return config11 },12 (error) => {13 return Promise.reject(error)14 }15)
การจัดการ Error
ตัว Error ที่ server ตอบกลับมาจะเข้า catch()
กรณีที่ไม่ใช่ HTTP 2xx โดยเราจะได้ response อยู่ในรูปแบบ error.response
ตัวอย่างเช่น
1axios.get('/some-api').catch(function (error) {2 if (error.response) {3 // The request was made and the server responded with a status code4 // that falls out of the range of 2xx5 console.log(error.response.data)6 console.log(error.response.status)7 console.log(error.response.headers)8 } else if (error.request) {9 // The request was made but no response was received10 // `error.request` is an instance of XMLHttpRequest in the browser and an instance of11 // http.ClientRequest in node.js12 console.log(error.request)13 } else {14 // Something happened in setting up the request that triggered an Error15 console.log('Error', error.message)16 }17 console.log(error.config)18})
หรือเราสามารถ ใช้ toJSON
เพื่อจัดการข้อมูลของ HTTP error ได้
1axios.get('/some-api').catch(function (error) {2 console.log(error.toJSON())3})
การ Cancel Request
เป็น feature ที่ค่อนข้างมีประโยชน์ กรณีที่เราทำการเรียก request ไปแล้ว และอยากยกเลิกมัน ก็สามารถทำได้ผ่านตัว AbortController
1const controller = new AbortController()2
3axios4 .get('/path/to/api', {5 signal: controller.signal6 })7 .then(function (response) {8 //...9 })10// cancel the request11controller.abort()
Credentials
Request ที่ยุ่งกับ cookies และ cross-site Access-Control
เราสามารถใช้ withCredentials
ได้ ตัวอย่าง
1import axios from 'axios';2
3const instance = axios.create({4 withCredentials: true5});6
7instance.get('/todos');8
9# หรือ10axios.post('/login', { email, password }, { withCredentials: true });
ทั้งหมดนี้ก็เป็นการใช้งาน axios แบบคร่าวๆ ซึ่งจริงๆ ก็ไม่มีอะไรมาก การ custom อื่นๆ หรือการประยุกต์ใช้งานในรายละเอียดอื่นๆ สามารถอ่านเพิ่มเติมได้ที่ Docs ของ axios ได้เลยครับ
Happy Coding ❤️
- Authors
-
Chai Phonbopit
เป็น Web Dev ในบริษัทแห่งหนึ่ง ทำงานมา 10 ปีกว่าๆ ด้วยภาษาและเทคโนโลยี เช่น JavaScript, Node.js, React, Vue และปัจจุบันกำลังสนใจในเรื่องของ Blockchain และ Crypto กำลังหัดเรียนภาษา Rust