วิธีการกำหนด Routes ของ React Router v6
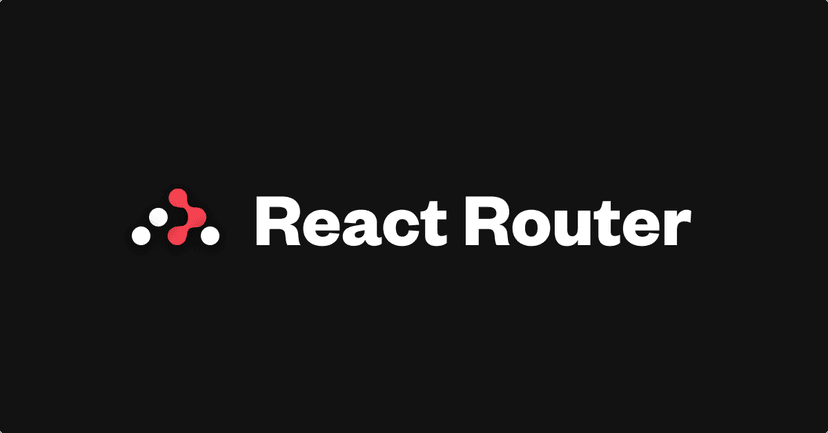
เนื่องจากช่วงนี้ได้กลับมาทบทวน React Router เพื่อนำมาทำเป็นเนื้อหาในคอร์สสอน React เบื้องต้น หลังจากที่ไม่ได้ใช้ Client Routing มานานมากๆ แล้ว (ปัจจุบัน แอพ React ทั้งหมด ผมใช้ Next.js ทั้งสิ้น)
วันนี้ลองไปนั่งอ่าน Doc ทบทวน ก็เลยนำมาเขียนเป็นบทความบันทึกเอาไว้ ถึงวิธีการกำหนด Routes ใน React Router ว่ามันทำท่าไหนได้บ้าง และทำอะไรได้บ้าง
ซึ่งจริงๆ แล้ว จากหน้าเว็บ เราสามารถอ่าน Tutorial และลองทำตามได้เลยนะ ใช้เวลาประมาณ 1 ชั่วโมง
การเลือก Router
ใน React Router v6 (v6.4) มันจะมีเรื่องของ Data API เข้ามาเกี่ยวข้องด้วย ฉะนั้น ก็มีมีวิธีการสร้าง Route แบบที่ใช้ data API และแบบที่ไม่ใช้ Data API นั่นเอง
BrowserRouter
วิธีแรก ขอเป็นแบบปกติ ที่เคยใช้ใน v4 / v5 หรือพวก reach router หรือ client side router ที่คุ้นๆกัน นั่นก็คือ ใช้แบบ BrowserRouter component
สมมติต้องการสร้าง Page ทั้งหมด
/
- หน้า Home Page (มี Layout เหมือนกัน คือ navbar)/about
- หน้า About/dashboard
- หน้า Dashboard/*
- ไม่ match ซัก url เลยก็จะโชว์ component NoMatch
วิธีนี้ก็คือ ตัว <BrowserRouter>
จะเก็บข้อมูล location จาก url ไว้ใน ตัว built-in history stack ปกติของ Browser (เวลากด back)
1import ReactDOM from 'react-dom/client'2import { BrowserRouter } from 'react-router-dom'3
4ReactDOM.createRoot(document.getElementById('root')!).render(5
6{' '}7
8<React.StrictMode>9 <BrowserRouter>10 <App />11 </BrowserRouter>12</React.StrictMode>13)
ในส่วนที่เราต้องการกำหนด Routing ก็จะใช้ <Routes>
และ <Route>
ตัวอย่างเช่นไฟล์ App.tsx
(Source Code)
1import { Routes, Route, Outlet, Link } from 'react-router-dom'2
3export default function App() {4 return (5 <div>6 <h1>Basic Example</h1>7
8 {/* Routes nest inside one another. Nested route paths build upon9 parent route paths, and nested route elements render inside10 parent route elements. See the note about <Outlet> below. */}11 <Routes>12 <Route path="/" element={<Layout />}>13 <Route index element={<Home />} />14 <Route path="about" element={<About />} />15 <Route path="dashboard" element={<Dashboard />} />16
17 {/* Using path="*"" means "match anything", so this route18 acts like a catch-all for URLs that we don't have explicit19 routes for. */}20 <Route path="*" element={<NoMatch />} />21 </Route>22 </Routes>23 </div>24 )25}26
27function Layout() {28 return (29 <div>30 {/* A "layout route" is a good place to put markup you want to31 share across all the pages on your site, like navigation. */}32 <nav>33 <ul>34 <li>35 <Link to="/">Home</Link>36 </li>37 <li>38 <Link to="/about">About</Link>39 </li>40 <li>41 <Link to="/dashboard">Dashboard</Link>42 </li>43 <li>44 <Link to="/nothing-here">Nothing Here</Link>45 </li>46 </ul>47 </nav>48
49 <hr />50
51 {/* An <Outlet> renders whatever child route is currently active,52 so you can think about this <Outlet> as a placeholder for53 the child routes we defined above. */}54 <Outlet />55 </div>56 )57}58
59function Home() {60 return (61 <div>62 <h2>Home</h2>63 </div>64 )65}66
67function About() {68 return (69 <div>70 <h2>About</h2>71 </div>72 )73}74
75function Dashboard() {76 return (77 <div>78 <h2>Dashboard</h2>79 </div>80 )81}82
83function NoMatch() {84 return (85 <div>86 <h2>Nothing to see here!</h2>87 <p>88 <Link to="/">Go to the home page</Link>89 </p>90 </div>91 )92}
createBrowserRouter
การสร้าง Route แบบใช้ createBrowserRouter
จะลองรับพวก Data API พวก loaders, actions, fetchers ด้วย แต่ว่าขอไม่พูดถึงละกัน ใครอยากรู้ลองไปอ่าน Tutorial ดูได้ครับ
การสร้างแบบนี้ ก็คล้ายๆ แบบแรก เพราะจริงๆ แบบแรก มันก็จะแปลงเป็น Object ลักษณะคล้ายๆกันนี่แหละ
สร้าง Router ขึ้นมาก่อน โดยกำหนดเป็น object array ที่เราต้องการ กำหนด path, element หรือ children เหมือนกับ <Route>
component เลย
1import { createBrowserRouter } from 'react-router-dom'2
3const router = createBrowserRouter([4 {5 path: '/',6 element: <Layout />,7 children: [8 {9 path: '/',10 index: true,11 element: <Home />12 },13 {14 path: 'about',15 element: <About />16 },17 {18 path: 'dashboard',19 element: <Dashboard />20 },21 {22 path: '*',23 element: <NoMatch />24 }25 ]26 }27])
ส่วน Main.tsx
ก็แค่เปลี่ยนจาก BrowserRouter เป็น <RouterProvider>
แทน แบบนี้
1import { RouterProvider } from 'react-router-dom'2
3ReactDOM.createRoot(document.getElementById('root')!).render(4
5{' '}6
7<React.StrictMode>8 <RouterProvider router={router} />9</React.StrictMode>10)
ผลลัพธ์ที่ได้ก็จะเหมือนแบบแรก
ลองไปอ่านเพิ่มเติม ฝึกเล่น ฝึกลองเขียนกันดูนะครับ (แต่จริงๆ ส่วนตัวผมมองว่า รู้แค่หลักการและวิธีการใช้งานก็พอ โอกาสที่จะใช้งาน React Router ก็คงต้องเป็นงานเฉพาะ ที่ไม่ได้ต้องการเรื่อง SEO เท่าไหร่ อาจจะเป็นหน้า Dashboard หรือระบบภายในมากกว่า)
เพราะถ้าทำระบบปัจจุบัน มี SEO เน้น Server Side ด้วย ผมมองว่า ก็ไปทาง Next.js เลย เพราะสุดท้าย ถ้าเราไม่ต้องการ Server Side ตัว Next.js ก็ build แค่ static file ให้เป็น Client Side ก็ได้เช่นกัน
Happy Coding ❤️
- Authors
-
Chai Phonbopit
เป็น Web Dev ในบริษัทแห่งหนึ่ง ทำงานมา 10 ปีกว่าๆ ด้วยภาษาและเทคโนโลยี เช่น JavaScript, Node.js, React, Vue และปัจจุบันกำลังสนใจในเรื่องของ Blockchain และ Crypto กำลังหัดเรียนภาษา Rust