Fetch Data ใน React.js ทำยังไงบ้าง?
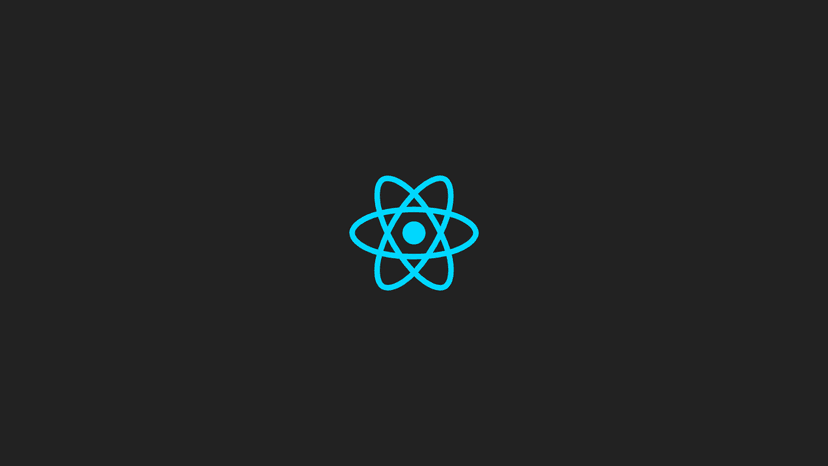
เราสามารถ fetch data ใน React.js ได้ท่าไหนบ้าง? มีทั้งการ fetch โดยไม่ต้องใช้ library อะไร และการใช้ library ที่ช่วยจัดการเรื่อง loading, caching หรือการ revalidating ต่างๆ ให้เรา การ fetch data มีท่าไหนบ้าง ไปดูกันครับ
ตัวอย่างจะใช้ API_URL
คือ
API_URL=https://jsonplaceholder.typicode.com/posts?_page=0&limit=9
1. การใช้ useEffect และ fetch/axios
วิธีที่ง่ายที่สุด คือการ fetch ใน useEffect()
เมื่อได้ข้อมูล response แล้ว ก็ทำการอัพเดท state เพื่อทำการ re-render component ใหม่
1import { useEffect, useState } from 'react'2import Posts from './posts'3
4import { API_URL } from '../constants'5
6const WithUseEffect = () => {7 const [posts, setPosts] = useState([])8
9 useEffect(() => {10 const getPosts = async () => {11 const res = await fetch(API_URL)12 const data = await res.json()13 setPosts(data)14 }15
16 getPosts()17 }, [])18
19 return (20 <>21 <h2>ตัวอย่าง fetch data ด้วย `useEffect`</h2>22 {posts.map((post) => (23 <p key={post.id}>{post.title}</p>24 ))}25 </>26 )27}28
29export default WithUseEffect
นอกจากนี้ เราอาจจะเพิ่ม state สำหรับ isLoading หรือ error กรณีที่ fetch data แล้วมีปัญหา เช่น อาจจะย้ายไปเป็น custom hooks ก็ได้เช่น
1const useFetchPosts = () => {2 const [data, setData] = useState([])3 const [isLoading, setIsLoading] = useState(false)4
5 useEffect(() => {6 const getPosts = async () => {7 const res = await fetch(API_URL)8 const data = await res.json()9 setPosts(data)10 }11
12 getPosts()13 }, [])14
15 return { data, isLoading }16}
2. ใช้ SWR
ตัว SWR เป็น React Hooks for Data fetching สร้างโดยทีมเดียวกับที่ทำ Next.js ข้อดีคือใช้งานง่าย มี caching / reuse ได้ ผมเคยเขียนตัวอย่าง SWR ไว้แล้ว สามารถอ่านรายละเอียดเพิ่มเติมได้
[React.js] ใช้ SWR เพื่อดึงข้อมูล API
อย่างแรก คือ สร้าง fetcher เป็น function ที่รับ args เป็น key และ return response data กลับไป
1const fetcher = (url) => fetch(url).then((r) => r.json())
ส่วนการ fetch ก็ง่ายๆ แบบนี้เลย
1import useSWR from 'swr'2import Posts from './posts'3
4import { API_URL } from '../constants'5
6const fetcher = (url) => fetch(url).then((r) => r.json())7
8const WithSWR = () => {9 const { data: posts, isLoading } = useSWR(API_URL, fetcher)10
11 if (isLoading) return <p>Loading...</p>12
13 return (14 <>15 <h2>ตัวอย่าง fetch data ด้วย `SWR`</h2>16 {posts.map((post) => (17 <p key={post.id}>{post.title}</p>18 ))}19 </>20 )21}22
23export default WithSWR
ข้อดีของ SWR คือ การ revadating หรือการคอย cached และ sync ข้อมูล api ให้เหมือนมัน realtime นั่นเอง ซึ่งถ้าเราใช้ useEffect()
และ fetch ปกติเราต้องจัดการ handle ตรงนี้เอง
3. การใช้ React Query
ตัวอย่างนี้จะเป็นการใช้งาน React Query ครับ การใช้ React Query คือ เราต้องกำหนด Provider โดยใช้ client เป็น queryClient
1import {2 QueryClient,3 QueryClientProvider,4} from '@tanstack/react-query'5
6const queryClient = new QueryClient()7
8<QueryClientProvider client={queryClient}>9 <App />10</QueryClientProvider>
การใช้งาน Query จะใช้ได้เฉพาะที่อยู่ภายใน <QueryClientProvider>
ตัวอย่างการ query คือต้องกำหนด
queryKey
เป็น key ที่เราต้องการqueryFn
เป็น function ที่เราจะใช้ในการ fetch ข้อมูล
1import { useQuery } from '@tanstack/react-query'2
3const { isLoading, data: posts } = useQuery({4 queryKey: ['repoData'],5 queryFn: () => fetch(API_URL).then((res) => res.json())6})
ตัวอย่างการ fetch data ด้วย React Query เต็มๆ ก็จะเป็นแบบนี้
1import { QueryClient, QueryClientProvider, useQuery } from '@tanstack/react-query'2
3import Posts from './posts'4import { API_URL } from '../constants'5
6const queryClient = new QueryClient()7
8function FetchPosts() {9 const { isLoading, data: posts } = useQuery({10 queryKey: ['repoData'],11 queryFn: () => fetch(API_URL).then((res) => res.json())12 })13
14 if (isLoading) return <p>Loading...</p>15
16 return (17 <>18 <h2>ตัวอย่าง `React Query`</h2>19 {posts.map((post) => (20 <p key={post.id}>{post.title}</p>21 ))}22 </>23 )24}25
26export default function WithReactQuery() {27 return (28 <QueryClientProvider client={queryClient}>29 <FetchPosts />30 </QueryClientProvider>31 )32}
สรุป
บทความนี้ก็เป็นตัวอย่างคร่าวๆ ในการ Fetch Data ในรูปแบบต่างๆ จะเห็นว่า แบบง่ายสุดคือ useEffect()
และแบบที่ใช้ทั้ง SWR หรือ React Query ทั้งสอง ก็คือเหมือนเป็นตัวช่วย อำนวยความสะดวก มี caching / revalidating หรือการจัดการ state loading, error ต่างๆ ให้เรา เพราะการ fetch เบื้องหลังยังไงก็ต้องใช้ fetch หรือ axios
Source Code ในบทความนี้
References
- Authors
-
Chai Phonbopit
เป็น Web Dev ในบริษัทแห่งหนึ่ง ทำงานมา 10 ปีกว่าๆ ด้วยภาษาและเทคโนโลยี เช่น JavaScript, Node.js, React, Vue และปัจจุบันกำลังสนใจในเรื่องของ Blockchain และ Crypto กำลังหัดเรียนภาษา Rust